Learn how to make an HTTP request in Javascript with our easy-to-follow step-by-step guide. We will provide you with all the information you need to make your request easily.
How to Make an HTTP Request in Javascript
HTTP requests are a crucial part of web development and have become an important skill to master. They are used to retrieve data from a remote server, and they are the foundation of AJAX (Asynchronous JavaScript and XML).
With the help of JavaScript, you can easily make an HTTP request that could be used to access data from a web server or even a third-party API.
What is an HTTP Request?
HTTP (Hypertext Transfer Protocol) is the underlying protocol used by the World Wide Web. It defines how messages are formatted and transmitted, and what actions web servers and browsers should take in response to various commands.
An HTTP request is sent by the client to the server to retrieve a particular resource. The request is composed of a request line, headers, and body (optional).
The request line includes the HTTP method, which defines the action to be taken, and the requested URL.
Headers provide additional information about the request, such as the type of content being sent and the authentication credentials. The body may contain further data, such as form data, which is sent along with the request.
How to Make an HTTP Request in JavaScript
Making an HTTP request in JavaScript is easy and straightforward. The XMLHttpRequest object is used to make HTTP requests in JavaScript.
It allows you to send and receive data from a remote server.
Step 1: Create an XMLHttpRequest Object
The first step is to create an XMLHttpRequest object. This object is used to make the actual request, and it contains all the necessary information and methods.
var xhr = new XMLHttpRequest();
Step 2: Open a Connection
Once the XMLHttpRequest object is created, you need to open a connection. This is done using the open() method of the object. The open() method takes three parameters: the HTTP method, the requested URL, and a Boolean value that indicates whether the request is asynchronous or not.
xhr.open('GET', 'https://example.com/data.json', true);
Step 3: Send the Request
The next step is to send the request. This is done using the send() method of the XMLHttpRequest object. The send() method takes an optional parameter, which is used to send data along with the request.
xhr.send();
Step 4: Process the Response
The final step is to process the response from the server. This is done using the onreadystatechange event handler of the XMLHttpRequest object. The onreadystatechange event is fired when the readyState property of the object changes. The readyState property can have one of the following values:
- 0: The request is not initialized
- 1: The request has been set up
- 2: The request has been sent
- 3: The request is in process
- 4: The request is complete
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
// Process the response
}
};
READ MORE: 10 EASY STEPS TO IMPROVE WORDPRESS SEO FOR YOUR SITE
To make an HTTP request in JavaScript, you can use the XMLHttpRequest object or the more modern fetch() function.
Here’s an example of how to use XMLHttpRequest to make a GET request to a server:
const xhr = new XMLHttpRequest();
xhr.responseType = 'json';
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
console.log(xhr.response);
}
}
xhr.open('GET', 'https://example.com/api/endpoint');
xhr.send();
READ MORE: HOW TO REMOVE WORDPRESS SEO ERRORS
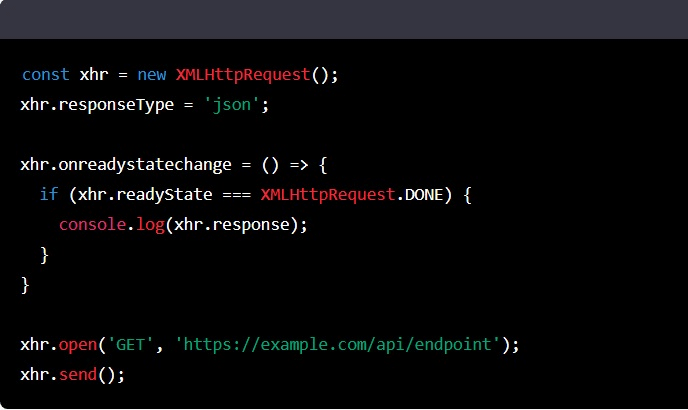
Here’s an example of how to use fetch() to make a GET request to a server:
fetch('https://example.com/api/endpoint')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));

Both XMLHttpRequest and fetch() can be used to make other types of HTTP requests, such as POST, PUT, and DELETE, by setting the method property in the options object passed to open() for XMLHttpRequest, or the method option for fetch().
Bottom Line
Making an HTTP request in JavaScript is easy and straightforward. You can use the XMLHttpRequest object to make the request and the onreadystatechange event handler to process the response.
With the help of this tutorial, you should now be able to make HTTP requests in JavaScript with ease.